BulletSpan
public
class
BulletSpan
extends Object
implements
LeadingMarginSpan,
ParcelableSpan
java.lang.Object | |
↳ | android.text.style.BulletSpan |
A span which styles paragraphs as bullet points (respecting layout direction).
BulletSpans must be attached from the first character to the last character of a single paragraph, otherwise the bullet point will not be displayed but the first paragraph encountered will have a leading margin.
BulletSpans allow configuring the following elements:
- gap width - the distance, in pixels, between the bullet point and the paragraph. Default value is 2px.
- color - the bullet point color. By default, the bullet point color is 0 - no color, so it uses the TextView's text color.
- bullet radius - the radius, in pixels, of the bullet point. Default value is 4px.
SpannableString string = new SpannableString("Text with\nBullet point");
string.setSpan(new BulletSpan(), 10, 22, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);
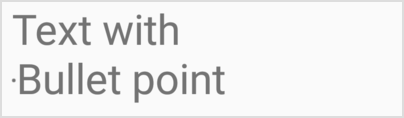
To construct a BulletSpan with a gap width of 40px, green bullet point and bullet radius of 20px:
SpannableString string = new SpannableString("Text with\nBullet point");
string.setSpan(new BulletSpan(40, color, 20), 10, 22, Spanned.SPAN_EXCLUSIVE_EXCLUSIVE);

Summary
Constants | |
---|---|
int |
STANDARD_GAP_WIDTH
|
Inherited constants |
---|
Public constructors | |
---|---|
BulletSpan()
Creates a |
|
BulletSpan(int gapWidth)
Creates a |
|
BulletSpan(int gapWidth, int color)
Creates a |
|
BulletSpan(int gapWidth, int color, int bulletRadius)
Creates a |
|
BulletSpan(Parcel src)
Creates a |
Public methods | |
---|---|
int
|
describeContents()
Describe the kinds of special objects contained in this Parcelable instance's marshaled representation. |
void
|
drawLeadingMargin(Canvas canvas, Paint paint, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. |
int
|
getBulletRadius()
Get the radius, in pixels, of the bullet point. |
int
|
getColor()
Get the bullet point color. |
int
|
getGapWidth()
Get the distance, in pixels, between the bullet point and the paragraph. |
int
|
getLeadingMargin(boolean first)
Returns the amount by which to adjust the leading margin. |
int
|
getSpanTypeId()
Return a special type identifier for this span class. |
String
|
toString()
Returns a string representation of the object. |
void
|
writeToParcel(Parcel dest, int flags)
Flatten this object in to a Parcel. |
Inherited methods | |
---|---|
Constants
STANDARD_GAP_WIDTH
public static final int STANDARD_GAP_WIDTH
Constant Value: 2 (0x00000002)
Public constructors
BulletSpan
public BulletSpan (int gapWidth)
Creates a BulletSpan
based on a gap width
Parameters | |
---|---|
gapWidth |
int : the distance, in pixels, between the bullet point and the paragraph. |
BulletSpan
public BulletSpan (int gapWidth, int color)
Creates a BulletSpan
based on a gap width and a color integer.
Parameters | |
---|---|
gapWidth |
int : the distance, in pixels, between the bullet point and the paragraph. |
color |
int : the bullet point color, as a color integer |
See also:
BulletSpan
public BulletSpan (int gapWidth, int color, int bulletRadius)
Creates a BulletSpan
based on a gap width and a color integer.
Parameters | |
---|---|
gapWidth |
int : the distance, in pixels, between the bullet point and the paragraph. |
color |
int : the bullet point color, as a color integer. |
bulletRadius |
int : the radius of the bullet point, in pixels.
Value is 0 or greater |
See also:
BulletSpan
public BulletSpan (Parcel src)
Creates a BulletSpan
from a parcel.
Parameters | |
---|---|
src |
Parcel : This value cannot be null . |
Public methods
describeContents
public int describeContents ()
Describe the kinds of special objects contained in this Parcelable
instance's marshaled representation. For example, if the object will
include a file descriptor in the output of writeToParcel(android.os.Parcel, int)
,
the return value of this method must include the
CONTENTS_FILE_DESCRIPTOR
bit.
Returns | |
---|---|
int |
a bitmask indicating the set of special object types marshaled
by this Parcelable object instance.
Value is either 0 or CONTENTS_FILE_DESCRIPTOR |
drawLeadingMargin
public void drawLeadingMargin (Canvas canvas, Paint paint, int x, int dir, int top, int baseline, int bottom, CharSequence text, int start, int end, boolean first, Layout layout)
Renders the leading margin. This is called before the margin has been
adjusted by the value returned by getLeadingMargin(boolean)
.
Parameters | |
---|---|
canvas |
Canvas : This value cannot be null . |
paint |
Paint : This value cannot be null . |
x |
int : the current position of the margin |
dir |
int : the base direction of the paragraph; if negative, the margin
is to the right of the text, otherwise it is to the left. |
top |
int : the top of the line |
baseline |
int : the baseline of the line |
bottom |
int : the bottom of the line |
text |
CharSequence : This value cannot be null . |
start |
int : the start of the line |
end |
int : the end of the line |
first |
boolean : true if this is the first line of its paragraph |
layout |
Layout : This value may be null . |
getBulletRadius
public int getBulletRadius ()
Get the radius, in pixels, of the bullet point.
Returns | |
---|---|
int |
the radius, in pixels, of the bullet point. |
getColor
public int getColor ()
Get the bullet point color.
Returns | |
---|---|
int |
the bullet point color |
getGapWidth
public int getGapWidth ()
Get the distance, in pixels, between the bullet point and the paragraph.
Returns | |
---|---|
int |
the distance, in pixels, between the bullet point and the paragraph. |
getLeadingMargin
public int getLeadingMargin (boolean first)
Returns the amount by which to adjust the leading margin. Positive values move away from the leading edge of the paragraph, negative values move towards it.
Parameters | |
---|---|
first |
boolean : true if the request is for the first line of a paragraph,
false for subsequent lines |
Returns | |
---|---|
int |
the offset for the margin. |
getSpanTypeId
public int getSpanTypeId ()
Return a special type identifier for this span class.
Returns | |
---|---|
int |
toString
public String toString ()
Returns a string representation of the object.
Returns | |
---|---|
String |
a string representation of the object. |
writeToParcel
public void writeToParcel (Parcel dest, int flags)
Flatten this object in to a Parcel.
Parameters | |
---|---|
dest |
Parcel : This value cannot be null . |
flags |
int : Additional flags about how the object should be written.
May be 0 or Parcelable.PARCELABLE_WRITE_RETURN_VALUE .
Value is either 0 or a combination of Parcelable.PARCELABLE_WRITE_RETURN_VALUE , and android.os.Parcelable.PARCELABLE_ELIDE_DUPLICATES |